Understanding Python Functions: A Key Programming Concept
Python functions are blocks of reusable and modular code that perform specific tasks. They contribute significantly to the organization and maintainability of code, making it more manageable and efficient. This article will explore various types of Python functions and their applications, with a focus on the main keyword “what are python functions.”
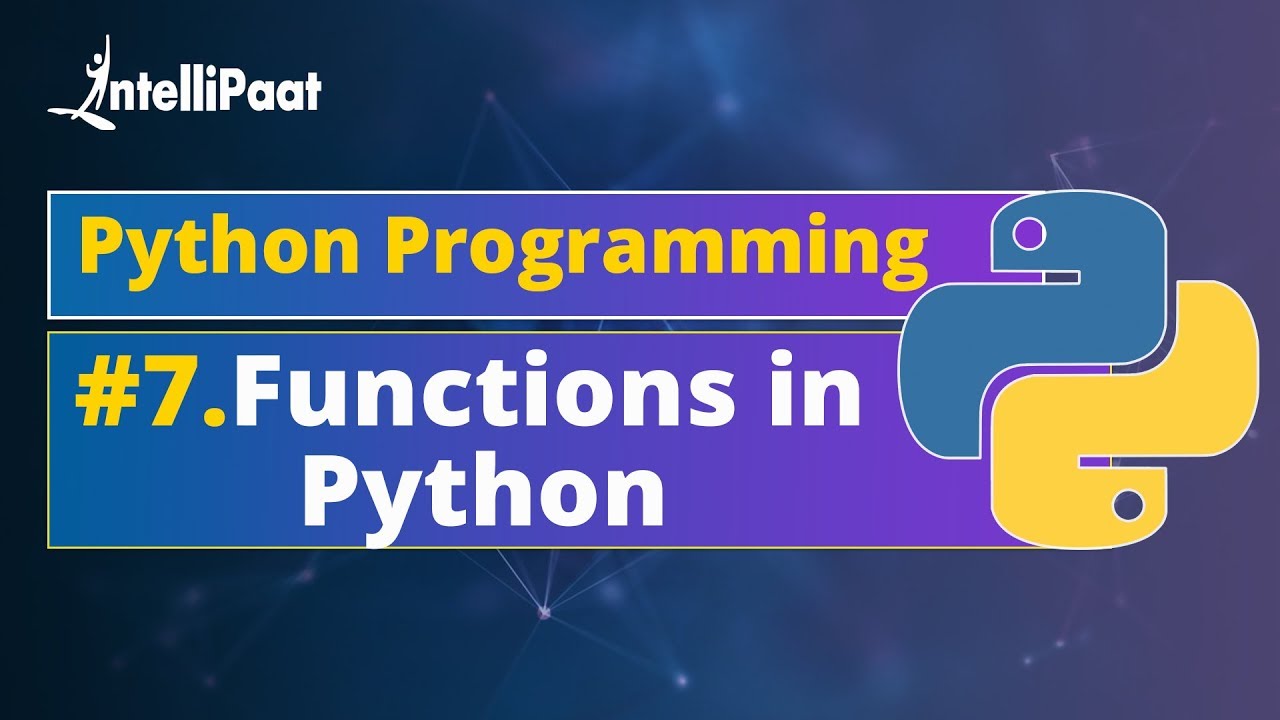
Basic Python Functions: Definitions and Syntax
To define a Python function, you must use the “def” keyword followed by the function name, parentheses containing input parameters, and a colon. The code block for the function is indented below the function definition. Here’s the basic structure:
def function_name(parameters): # Function code block
For example, a simple function that adds two numbers and returns the result can be defined as:
def add_two_numbers(num1, num2): return num1 + num2
When defining Python functions, it is essential to consider input parameters and return values. Input parameters are the data passed to the function, while return values are the output provided by the function. In the above example, “num1” and “num2” are input parameters, and the function returns their sum.

Function Arguments in Python: Various Input Mechanisms
Python functions support several ways to pass arguments, enhancing their flexibility and usability. These mechanisms include positional arguments, keyword arguments, default arguments, and variable-length arguments.
Positional Arguments
Positional arguments are passed to functions based on their position in the argument list. The order of the arguments matters when using positional arguments. Here’s an example:
def greet(name, greeting): return f"{greeting}, {name}!" print(greet("Alice", "Hello")) # Output: Hello, Alice!
Keyword Arguments
Keyword arguments, also known as named arguments, are passed to functions using their names rather than their positions. This allows for better readability and reduces the risk of passing arguments in the wrong order. Keyword arguments are defined after the positional arguments in the function definition.
def greet(name, greeting): return f"{greeting}, {name}!" print(greet(greeting="Hello", name="Alice")) # Output: Hello, Alice!
Default Arguments
Default arguments are arguments that take a default value if no value is provided when calling the function. Default arguments are defined after the positional and keyword arguments in the function definition.
def greet(name="World", greeting="Hello"): return f"{greeting}, {name}!" print(greet()) # Output: Hello, World!
print(greet("Alice")) # Output: Hello, Alice!
print(greet("Alice", "Good morning")) # Output: Good morning, Alice!
Variable-Length Arguments
Variable-length arguments, also known as variable positional arguments, allow a function to accept any number of positional arguments. Variable-length arguments are defined using the “*” symbol followed by the argument name in the function definition. The arguments are then passed as a tuple.
def sum_numbers(*numbers): return sum(numbers) print(sum_numbers(1, 2, 3, 4, 5)) # Output: 15

Types of Python Functions: Regular, Lambda, and Anonymous Functions
Python offers three main types of functions: regular functions, lambda functions, and anonymous functions. Each type has unique characteristics, use cases, and syntax.
Regular Functions
Regular functions, also known as defined functions, are the most common type of function in Python. They are defined using the “def” keyword, followed by the function name, input parameters, and a colon. The code block for the function is indented below the function definition. Here’s the basic structure:
def function_name(parameters): # Function code block
For example, a simple regular function that adds two numbers and returns the result can be defined as:
def add_two_numbers(num1, num2): return num1 + num2
Lambda Functions
Lambda functions, also known as anonymous functions, are small, single-use functions defined using the “lambda” keyword. They can have any number of arguments but only one expression. Lambda functions are typically used in situations where a full regular function is not required, such as when passing a function as an argument to another function.
add = lambda num1, num2: num1 + num2 print(add(3, 4)) # Output: 7
Anonymous Functions
Anonymous functions are similar to lambda functions but can have multiple expressions and are defined using the “def” keyword. They are rarely used in Python, as lambda functions are more concise and better suited for single-use functions.
add = def(num1, num2): return num1 + num2 print(add(3, 4)) # Output: 7
Anonymous functions are not recommended for general use, as they can lead to confusion and make code less readable. Lambda functions are preferred when a small, single-use function is required.
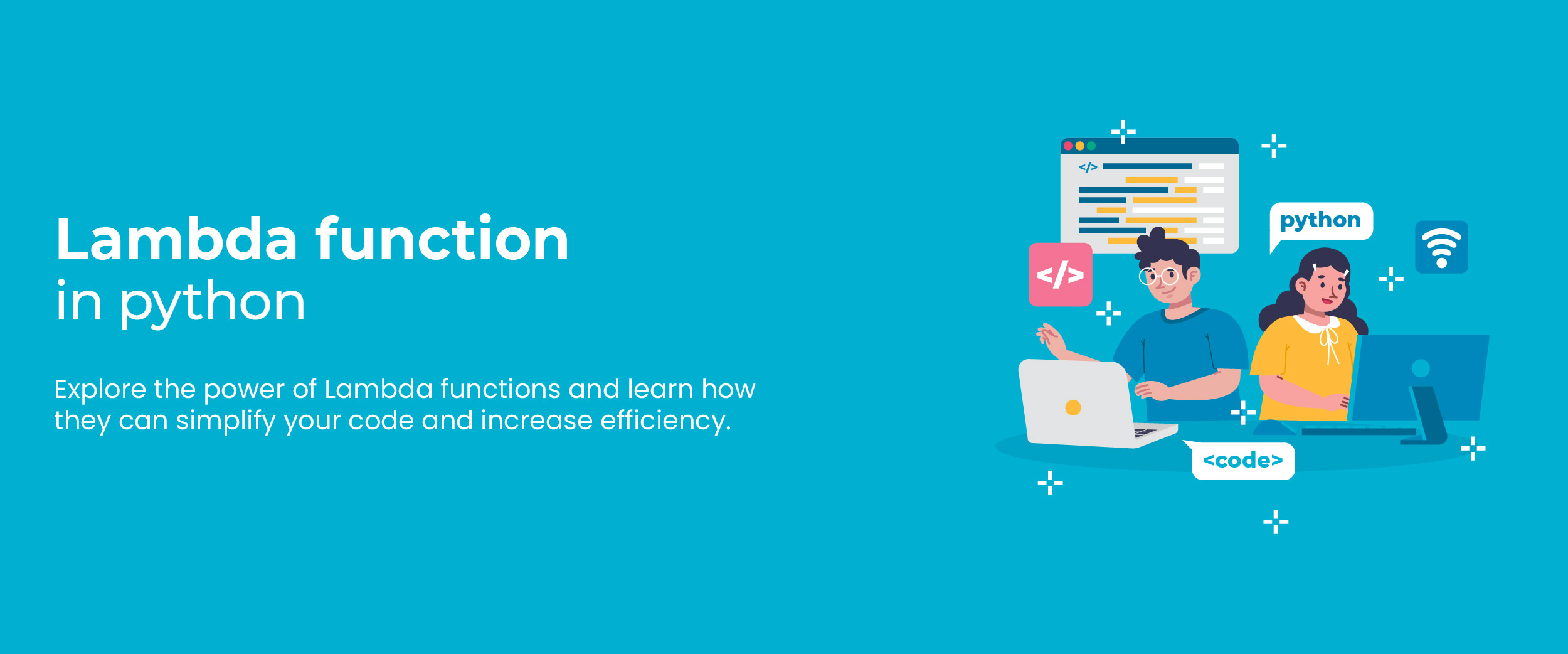
Anonymous Functions in Python: Lambda Functions
Lambda functions, also known as anonymous functions, are small, single-use functions defined using the “lambda” keyword. They can have any number of arguments but only one expression. Lambda functions are typically used in situations where a full regular function is not required, such as when passing a function as an argument to another function. The general syntax for a lambda function is:
lambda arguments: expression
Lambda functions are particularly useful when working with higher-order functions, such as map(), filter(), and reduce(). These functions accept other functions as arguments, allowing you to perform operations on iterable objects like lists and dictionaries.
Example: Using Lambda Functions with the map() Function
The map() function applies a given function to each item in an iterable and returns a new iterable with the results. In this example, we’ll use a lambda function to convert a list of strings to uppercase:
numbers = [1, 2, 3, 4, 5]
Using a lambda function with the map() function
uppercase_numbers = map(lambda x: str(x).upper(), numbers)
Convert the map object to a list to print the results
print(list(uppercase_numbers)) # Output: ['1', '2', '3', '4', '5']
Example: Using Lambda Functions with the filter() Function
The filter() function filters elements in an iterable based on a given function that returns a boolean value. In this example, we’ll use a lambda function to filter out odd numbers from a list:
numbers = [1, 2, 3, 4, 5]
Using a lambda function with the filter() function
odd_numbers = filter(lambda x: x % 2 != 0, numbers)
Convert the filter object to a list to print the results
print(list(odd_numbers)) # Output: [1, 3, 5]
Lambda functions are a powerful and concise way to define simple, single-use functions in Python. By understanding how to use lambda functions, you can enhance your ability to write clean, efficient, and maintainable code.

How to Define and Use Anonymous Functions in Python
Anonymous functions, or lambda functions, are a concise way to define simple, single-use functions in Python. They are defined using the “lambda” keyword and have the following general syntax:
lambda arguments: expression
Lambda functions can be particularly useful when working with higher-order functions, such as map(), filter(), and reduce(). These functions accept other functions as arguments, allowing you to perform operations on iterable objects like lists and dictionaries.
Example: Defining and Using a Lambda Function
In this example, we’ll define a lambda function that calculates the square of a number and then use it to calculate the squares of several numbers:
# Define a lambda function that calculates the square of a number square = lambda x: x ** 2
Use the lambda function to calculate the squares of several numbers
squares_list = [square(num) for num in range(1, 6)]
print(squares_list) # Output: [1, 4, 9, 16, 25]
Advantages and Limitations of Lambda Functions
Lambda functions offer several advantages, including:
- Conciseness: Lambda functions are a quick and easy way to define simple functions without the need for a full function definition.
- Anonymous: Lambda functions do not have a name, which can make them useful when you need a function for a single, specific purpose.
- Compatibility: Lambda functions are compatible with higher-order functions like map(), filter(), and reduce(), making them a powerful tool for functional programming in Python.
However, lambda functions also have limitations:
- Limited scope: Lambda functions can only contain a single expression, which can limit their usefulness for more complex operations.
- Less readable: Lambda functions can be less readable and more difficult to understand than regular functions, especially for developers unfamiliar with this syntax.
When deciding whether to use a lambda function or a regular function, consider the complexity of the operation, the need for a named function, and the compatibility with higher-order functions. By understanding how to define and use anonymous functions in Python, you can write more concise and efficient code.

Python Functions and Recursion: Defining Functions That Call Themselves
Recursion is a programming concept where a function calls itself to solve a problem by breaking it down into smaller, more manageable sub-problems. Recursive functions can be particularly useful for solving problems that involve repetition, tree structures, or complex data manipulation.
Example: A Simple Recursive Function to Calculate Factorials
In this example, we’ll define a recursive function to calculate the factorial of a number:
def factorial(n):
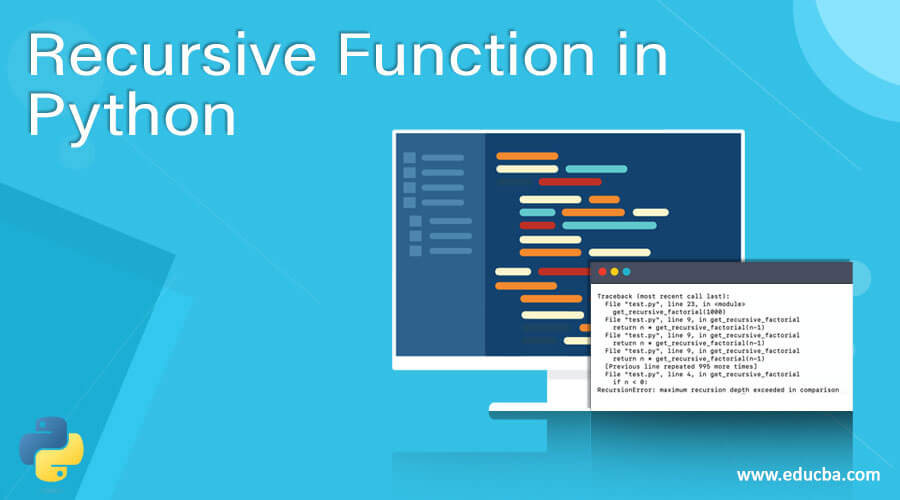
Scope of Python Functions: Understanding Local and Global Variables
When working with Python functions, it’s essential to understand the concept of variable scope, which refers to the visibility and accessibility of variables within a program. Python has two types of variable scopes: local and global.
Local Variables
Local variables are variables that are defined inside a function and are only accessible within that function’s scope. Once the function finishes executing, the local variables are destroyed.
def add_numbers(a, b): result = a + b print(f"Inside the function: {result}") add_numbers(3, 5) # Output: Inside the function: 8
print(f"Outside the function: {result}") # NameError: name 'result' is not defined
Global Variables
Global variables are variables that are defined outside of any function and are accessible from any part of the program, including within functions. To modify a global variable within a function, you need to use the “global” keyword.
result = 0 def add_numbers(a, b):
global result
result = a + b
print(f"Inside the function: {result}")
add_numbers(3, 5) # Output: Inside the function: 8
print(f"Outside the function: {result}") # Output: Outside the function: 8
Best Practices for Managing Variable Scope
To ensure proper variable scope management in your Python functions, follow these best practices:
- Limit the use of global variables: Global variables can make code harder to understand, debug, and maintain. Instead, try to pass necessary data as function arguments and return values.
- Use local variables for temporary storage: Local variables are ideal for storing intermediate results or temporary data within a function.
- Be mindful of variable shadowing: Variable shadowing occurs when a local variable has the same name as a global variable. This can lead to confusion and unexpected behavior. To avoid variable shadowing, choose unique variable names for each scope.
By understanding the scope of Python functions and how to manage local and global variables, you can write more organized, maintainable, and bug-free code.