What is AKS and Why Use Terraform?
Azure Kubernetes Service (AKS) is a managed container orchestration service provided by Microsoft Azure. AKS simplifies the deployment, scaling, and management of Kubernetes clusters, allowing developers to focus on building and deploying applications. Terraform, on the other hand, is an open-source Infrastructure as Code (IaC) tool that enables developers to define, provision, and manage infrastructure resources in a consistent and efficient manner.
Using Terraform with AKS offers several benefits, including version control for infrastructure, ease of collaboration, and the ability to automate infrastructure provisioning. By using Terraform to manage AKS clusters, developers can ensure that their infrastructure is consistently deployed and configured, reducing the risk of errors and inconsistencies. Additionally, Terraform’s modular and reusable syntax makes it easy to manage complex infrastructure environments, allowing developers to scale and upgrade their AKS clusters as needed.
In this comprehensive guide, we will explore the necessary prerequisites for using Terraform with AKS, provide a step-by-step guide on how to create an AKS cluster using Terraform, and discuss best practices for securing, scaling, and monitoring your AKS cluster. By the end of this article, you will have a solid understanding of how to use Terraform to manage your AKS cluster and be equipped with the knowledge and skills to effectively deploy and manage containerized applications in the cloud.
Prerequisites for Using Terraform with AKS
Before you can start using Terraform with AKS, there are a few prerequisites that you need to meet. First and foremost, you need an active Azure account. If you don’t already have one, you can sign up for a free trial on the Microsoft Azure website. Once you have an Azure account, you will also need to install the Azure CLI on your local machine. The Azure CLI is a command-line tool that enables you to create and manage Azure resources from the command line.
In addition to an active Azure account and the Azure CLI, you will also need to install Terraform on your local machine. Terraform is available for Windows, Mac, and Linux, and can be downloaded from the official Terraform website. Once you have downloaded and installed Terraform, you can verify that it is working properly by running the terraform
command from the command line. If Terraform is installed correctly, you should see a list of available Terraform commands.
Before you can start using Terraform with AKS, you will also need to create an Azure service principal. A service principal is a security identity that you can use to authenticate to Azure services, such as AKS. To create a service principal, you can use the Azure CLI command az ad sp create-for-rbac
. This command will create a new service principal and output the necessary credentials, including the client ID, tenant ID, and client secret. You will need to provide these credentials to Terraform later on, when you configure your AKS provider.
Once you have met all of the prerequisites, you are ready to start using Terraform with AKS. In the next section, we will provide a detailed, step-by-step guide on how to create an AKS cluster using Terraform, including code snippets and screenshots.
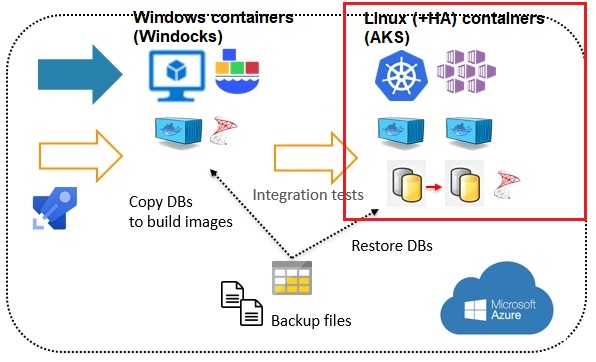
Creating an AKS Cluster with Terraform: Step-by-Step
Now that you have met all of the prerequisites for using Terraform with AKS, it’s time to create your AKS cluster. In this section, we will provide a detailed, step-by-step guide on how to create an AKS cluster using Terraform, including code snippets and screenshots.
The first step in creating an AKS cluster with Terraform is to define your infrastructure as code. To do this, you will need to create a Terraform configuration file that describes the resources you want to create. In this example, we will create a simple AKS cluster with a single node pool. Here is the Terraform configuration file we will be using:
provider "azurerm" { features {} } resource "azurerm_resource_group" "example" { name = "example-resources" location = "East US" } resource "azurerm_kubernetes_cluster" "example" { name = "example-aks" location = azurerm\_resource\_group.example.location resource\_group\_name = azurerm\_resource\_group.example.name dns\_prefix = "exampleaks" default\_node\_pool { name = "default" node\_count = 1 vm\_size = "Standard\_DS2\_v2" } identity { type = "SystemAssigned" } }
This configuration file defines an Azure resource group and an AKS cluster. The AKS cluster has a single node pool with a default node count of 1 and a VM size of Standard\_DS2\_v2. It also has a system-assigned identity, which is required for AKS clusters in Azure.
To apply this configuration file with Terraform, you can use the terraform apply
command. This command will prompt you to confirm that you want to create the resources described in the configuration file. Once you confirm, Terraform will create the resources in your Azure subscription.
After the resources have been created, you can verify that your AKS cluster is running by using the kubectl get nodes
command. This command will display a list of nodes in your cluster, including their status and IP addresses.
Congratulations! You have successfully created an AKS cluster using Terraform. In the next section, we will explain how to configure network settings for your AKS cluster, such as setting up a virtual network, subnets, and load balancers.
Configuring Network Settings for Your AKS Cluster
Once you have created your AKS cluster using Terraform, the next step is to configure network settings for your cluster. In this section, we will explain how to set up a virtual network, subnets, and load balancers for your AKS cluster.
Setting Up a Virtual Network
To set up a virtual network for your AKS cluster, you will need to create a new virtual network resource in your Azure subscription. Here is an example Terraform configuration file that creates a virtual network with a single subnet:
resource "azurerm\_virtual\_network" "example" { name = "example-vnet" resource\_group\_name = azurerm\_resource\_group.example.name location = azurerm\_resource\_group.example.location address\_space = ["10.0.0.0/16"] subnet { name = "example-subnet" address\_prefixes = ["10.0.1.0/24"] } }
This configuration file creates a virtual network with the name example-vnet
and a single subnet with the name example-subnet
and an address prefix of 10.0.1.0/24
.
Setting Up Subnets
In addition to the default subnet, you can also create additional subnets for your AKS cluster. For example, you might want to create a separate subnet for your pods and another subnet for your services. Here is an example Terraform configuration file that creates two additional subnets for an AKS cluster:
resource "azurerm\_subnet" "example" { count = 2 name = "example-subnet-${count.index}" resource\_group\_name = azurerm\_resource\_group.example.name virtual\_network\_name = azurerm\_virtual\_network.example.name address\_prefixes = ["10.0.${count.index + 2}.0/24"] }
This configuration file creates two additional subnets, named example-subnet-0
and example-subnet-1
, with address prefixes of 10.0.2.0/24
and 10.0.3.0/24
, respectively.
Setting Up Load Balancers
To expose your AKS cluster to the internet, you will need to set up a load balancer. Here is an example Terraform configuration file that creates a load balancer for an AKS cluster:
resource "azurerm\_public\_ip" "example" { name = "example-public-ip" resource\_group\_name = azurerm\_resource\_group.example.name location = azurerm\_resource\_group.example.location allocation\_method = "Static" } resource "azurerm\_lb" "example" { name = "example-lb" resource\_group\_name = azurerm\_resource\_group.example.name location = azurerm\_resource\_group.example.location frontend\_ip\_configuration { name = "example-frontend-ip" public\_ip\_address\_id = azurerm\_public\_ip.example.id } } resource "azurerm\_lb\_backend\_address\_pool" "example" { name = "example-backend-pool" resource\_group\_name = azurerm\_resource\_group.example.name loadbalancer\_id = azurerm\_lb.example.id } resource "azurerm\_network\_interface\_backend\_address\_pool\_association" "example" { count = 3 network\_interface\_id = azurerm\_network\_interface.example[count.index].id ip\_configuration\_name = "example-ip-config-${count.index}" backend\_address\_pool\_id = azurerm\_lb\_backend\_address\_pool.example.id }
This configuration file creates a public IP address, a load balancer, a backend address pool, and associates the load balancer with the network interfaces of the nodes in your AKS cluster. This will enable traffic to flow to your AKS cluster from the internet.
In the next section, we will discuss how to secure your AKS cluster using RBAC (Role-Based Access Control) and network policies. This will help ensure that your cluster is protected against unauthorized access and malicious traffic.
Securing Your AKS Cluster with RBAC and Network Policies
Securing your AKS cluster is an essential step in ensuring the safety and integrity of your containerized applications. In this section, we will discuss how to secure your AKS cluster using RBAC (Role-Based Access Control) and network policies. We will also provide examples and best practices to help you get started.
Role-Based Access Control (RBAC)
Role-Based Access Control (RBAC) is a security feature in AKS that enables you to control who has access to your cluster and what permissions they have. With RBAC, you can create roles that define specific actions that can be performed on resources within your cluster. You can then assign these roles to users or groups, giving them the exact permissions they need to do their job.
Here is an example Terraform configuration file that creates an RBAC role and assigns it to a user:
resource "azurerm\_role\_definition" "example" { name = "example-role" scope = "/subscriptions/00000000-0000-0000-0000-000000000000" permissions { actions = [ "Microsoft.ContainerService/managedClusters/listClusterAdminCredential/action", ] not\_actions = [] } assignable\_scopes = [ "/subscriptions/00000000-0000-0000-0000-000000000000", ] } resource "azurerm\_role\_assignment" "example" { scope = azurerm\_role\_definition.example.scope role\_definition\_id = azurerm\_role\_definition.example.id principal\_id = "11111111-1111-1111-1111-111111111111" }
This configuration file creates a new role definition with the name example-role
and assigns it to the user with the principal ID 11111111-1111-1111-1111-111111111111
. The role definition includes the permission to list the cluster admin credentials for the AKS cluster in the specified subscription.
Network Policies
Network policies are a way to control the flow of traffic between pods in your AKS cluster. With network policies, you can define rules that allow or deny traffic based on labels, namespaces, and other criteria. This can help you prevent unwanted traffic from reaching your pods and improve the overall security of your cluster.
Here is an example Terraform configuration file that creates a network policy for an AKS cluster:
resource "azurerm\_kubernetes\_cluster" "example" { name = "example-aks" location = azurerm\_resource\_group.example.location resource\_group\_name = azurerm\_resource\_group.example.name dns\_prefix = "exampleaks" default\_node\_pool { name = "default" node\_count = 1 vm\_size = "Standard\_DS2\_v2" } network\_profile { network\_plugin = "azure" service\_cidr = "10.0.0.0/16" dns\_service\_ip = "10.0.0.10" docker\_bridge\_cidr = "172.17.0.1/16" } network\_policy { enabled = true } } resource "kubernetes\_network\_policy" "example" { metadata { name = "example-policy" } spec { pod\_selector { match\_labels = { app = "example-app" } } ingress { from { pod\_selector { match\_labels = { app = "example-frontend" } } } } } }
This configuration file creates a new AKS cluster with network policies enabled. It also creates a network policy named example-policy
that allows traffic to flow between pods with the label app: example-app
and pods with the label app: example-frontend
.
By using RBAC and network policies, you can significantly improve the security of your AKS cluster and protect your containerized applications from unauthorized access and malicious traffic.
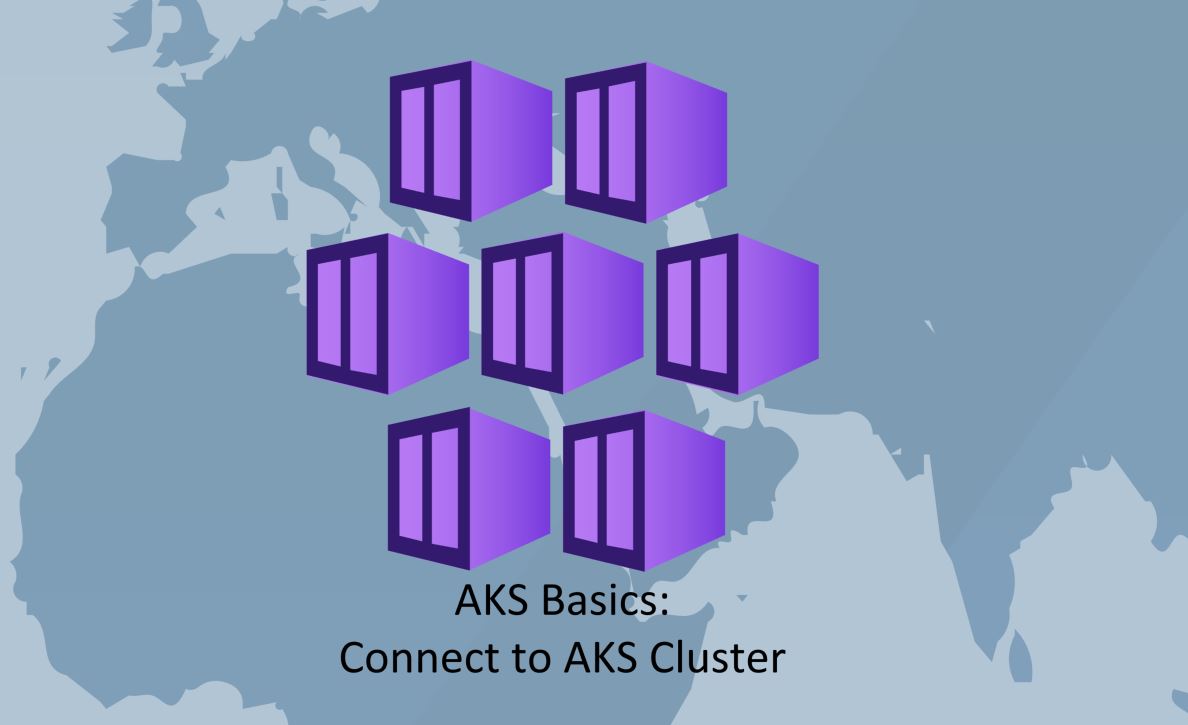
Scaling and Upgrading Your AKS Cluster with Terraform
As your needs change, you may need to scale or upgrade your AKS cluster. In this section, we will discuss how to use Terraform to scale and upgrade your AKS cluster, including how to add or remove nodes and upgrade Kubernetes versions.
Scaling Your AKS Cluster
To scale your AKS cluster, you can use the azurerm\_kubernetes\_cluster\_node\_pool
resource in Terraform. This resource allows you to add or remove nodes from your cluster, depending on your needs. Here is an example Terraform configuration file that scales an AKS cluster from 3 nodes to 5 nodes:
resource "azurerm\_kubernetes\_cluster" "example" { name = "example-aks" location = azurerm\_resource\_group.example.location resource\_group\_name = azurerm\_resource\_group.example.name dns\_prefix = "exampleaks" default\_node\_pool { name = "default" node\_count = 5 vm\_size = "Standard\_DS2\_v2" } network\_profile { network\_plugin = "azure" service\_cidr = "10.0.0.0/16" dns\_service\_ip = "10.0.0.10" docker\_bridge\_cidr = "172.17.0.1/16" } }
In this example, the node\_count
property of the default\_node\_pool
resource is set to 5, which will scale the cluster to 5 nodes. To scale the cluster back down to 3 nodes, you can simply change the node\_count
property to 3 and run terraform apply
again.
Upgrading Your AKS Cluster
To upgrade your AKS cluster, you can use the kubernetes\_cluster\_upgrade
resource in Terraform. This resource allows you to upgrade the Kubernetes version of your cluster to a newer version. Here is an example Terraform configuration file that upgrades an AKS cluster to the latest Kubernetes version:
resource "kubernetes\_cluster\_upgrade" "example" { cluster\_id = azurerm\_kubernetes\_cluster.example.id kubernetes\_version = data.azurerm\_kubernetes\_service\_versions.example.latest\_version } data "azurerm\_kubernetes\_service\_versions" "example" { location = azurerm\_resource\_group.example.location }
In this example, the kubernetes\_cluster\_upgrade
resource upgrades the AKS cluster specified by the cluster\_id
property to the latest Kubernetes version, as determined by the latest\_version
property of the azurerm\_kubernetes\_service\_versions
data source. To upgrade to a specific version, you can simply set the kubernetes\_version
property to the desired version.
By using Terraform to scale and upgrade your AKS cluster, you can ensure that your cluster is always running the latest version of Kubernetes and has the resources it needs to support your containerized applications.
Monitoring and Troubleshooting Your AKS Cluster
Monitoring and troubleshooting your AKS cluster is an important part of maintaining its health and availability. In this section, we will discuss how to use Terraform to monitor and troubleshoot your AKS cluster, including how to view logs, metrics, and diagnose issues.
Viewing Logs and Metrics
To view logs and metrics for your AKS cluster, you can use the azurerm\_monitor\_metric\_alert
and azurerm\_monitor\_log\_analytics\_workspace
resources in Terraform. These resources allow you to collect, analyze, and alert on metrics and logs from your AKS cluster. Here is an example Terraform configuration file that creates a log analytics workspace and a metric alert for an AKS cluster:
resource "azurerm\_monitor\_log\_analytics\_workspace" "example" { name = "example-workspace" location = azurerm\_resource\_group.example.location resource\_group\_name = azurerm\_resource\_group.example.name sku = "PerGB2018" } resource "azurerm\_monitor\_metric\_alert" "example" { name = "example-alert" resource\_group\_name = azurerm\_resource\_group.example.name scopes = [azurerm\_kubernetes\_cluster.example.id] description = "This alert will trigger when CPU usage exceeds 80%" severity = "3" frequency = "PT5M" time\_window = "PT15M" criteria { metric\_name = "Percentage CPU" metric\_resource\_id = azurerm\_kubernetes\_cluster.example.id aggregation = "Average" operator = "GreaterThan" threshold = "80" } action { action\_group\_id = azurerm\_monitor\_action\_group.example.id } } resource "azurerm\_monitor\_action\_group" "example" { name = "example-action-group" resource
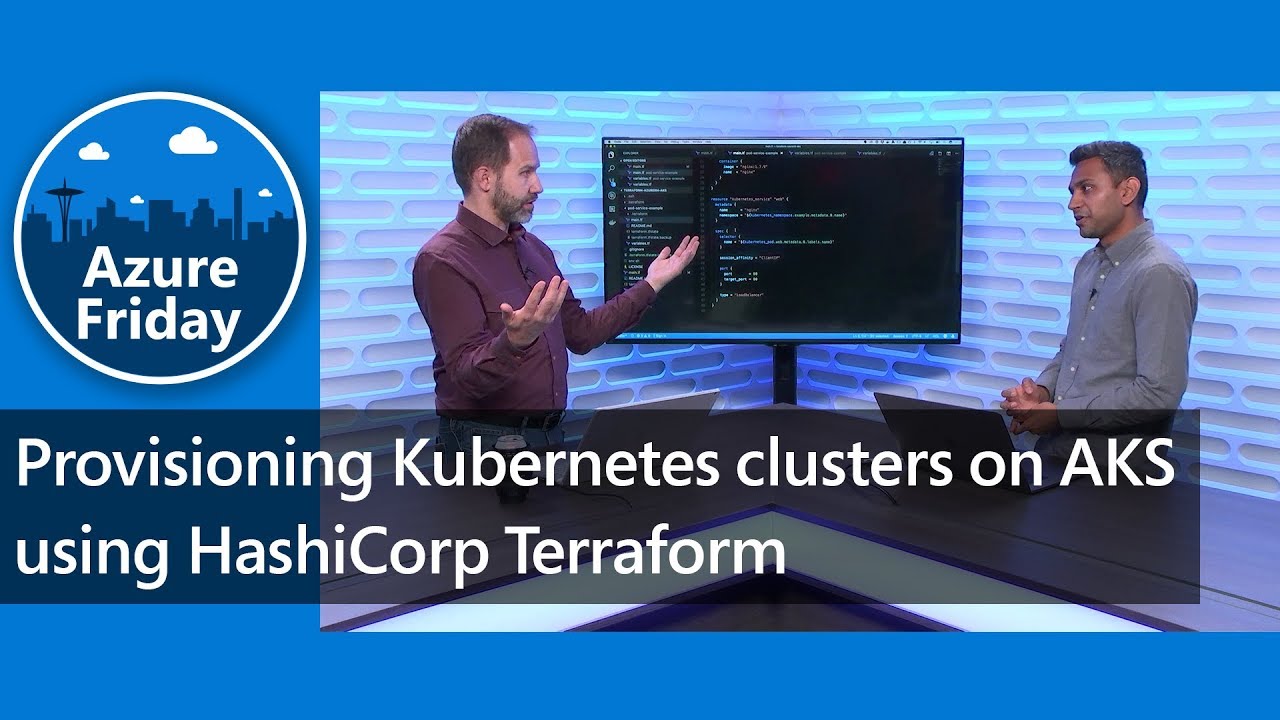
Best Practices for Using Terraform with AKS
Terraform is a powerful tool for deploying and managing AKS clusters, but it’s important to follow best practices to ensure that your infrastructure is stable, secure, and easy to maintain. In this section, we will discuss some best practices for using Terraform with AKS, including tips on code organization, testing, and collaboration.
Code Organization
When working with Terraform and AKS, it’s important to organize your code in a way that is easy to understand and maintain. Here are some tips for organizing your Terraform code:
- Use modules: Modules are reusable units of Terraform code that can be shared across different projects and teams. By using modules, you can reduce duplication and make your code more modular and maintainable.
- Use variables: Variables allow you to parameterize your Terraform code, making it easier to reuse and customize. Use variables to specify values that may change, such as the number of nodes in your AKS cluster or the Kubernetes version you want to use.
- Use outputs: Outputs allow you to extract values from your Terraform configuration and use them in other parts of your infrastructure. Use outputs to extract values such as the IP address of your load balancer or the name of your AKS cluster.
Testing
Testing is an important part of any infrastructure deployment, and Terraform makes it easy to test your infrastructure code. Here are some tips for testing your Terraform code:
- Use
terraform plan
: Theterraform plan
command allows you to preview the changes that Terraform will make to your infrastructure without actually applying them. Use this command to verify that your Terraform code is doing what you expect before you apply it. - Use
terraform validate
: Theterraform validate
command checks your Terraform code for syntax errors and other issues. Use this command to catch errors early in the development process. - Use integration tests: Integration tests allow you to test your Terraform code in a real-world environment. Use integration tests to verify that your infrastructure is working as expected and to catch any issues before they become production problems.
Collaboration
Collaboration is key to any successful infrastructure deployment, and Terraform makes it easy to collaborate with others. Here are some tips for collaborating with your team using Terraform:
- Use version control: Version control allows you to track changes to your Terraform code and collaborate with your team. Use a version control system such as Git to manage your Terraform code and collaborate with your team.
- Use workspace: Workspaces allow you to manage multiple environments with a single Terraform configuration. Use workspaces to manage different environments, such as development, staging, and production, and to collaborate with your team.
- Use remote state: Remote state allows you to store your Terraform state in a central location, making it easy for your team to access and collaborate on your infrastructure. Use a remote state backend such as Azure Storage or Amazon S3 to store your Terraform state and collaborate with your team.
By following these best practices, you can ensure that your AKS cluster is stable, secure, and easy to maintain. Happy deploying!
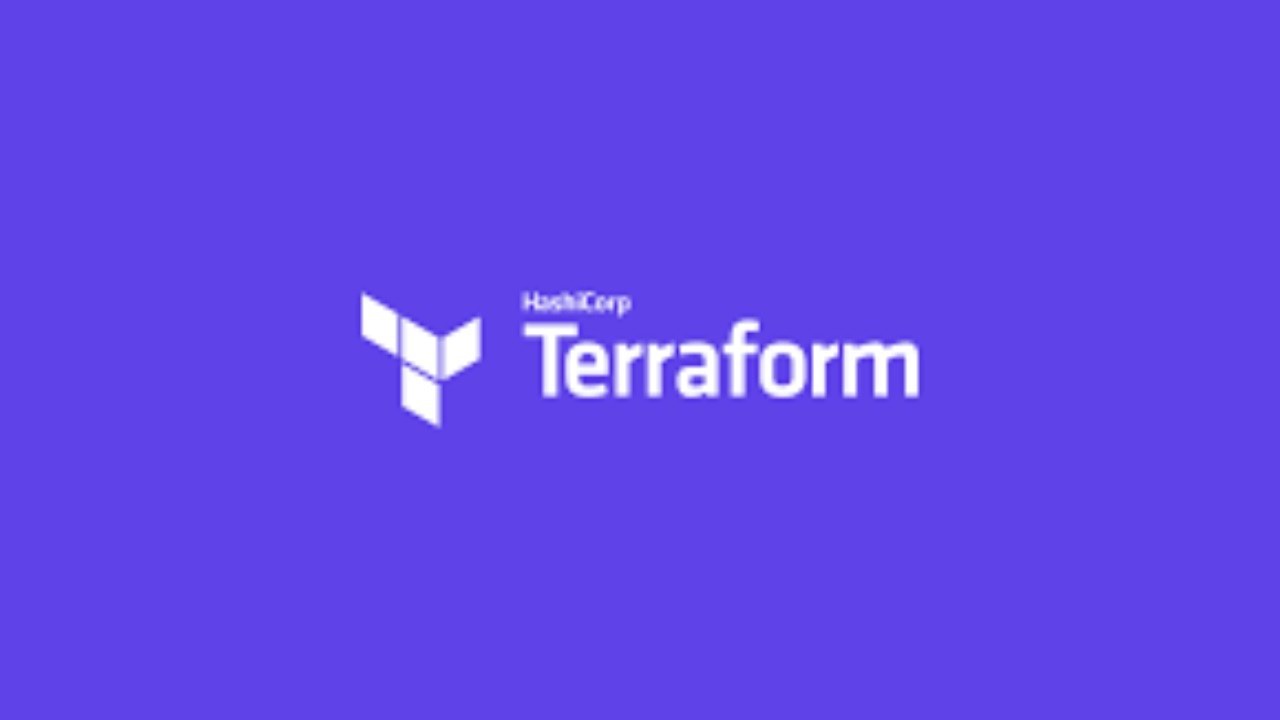